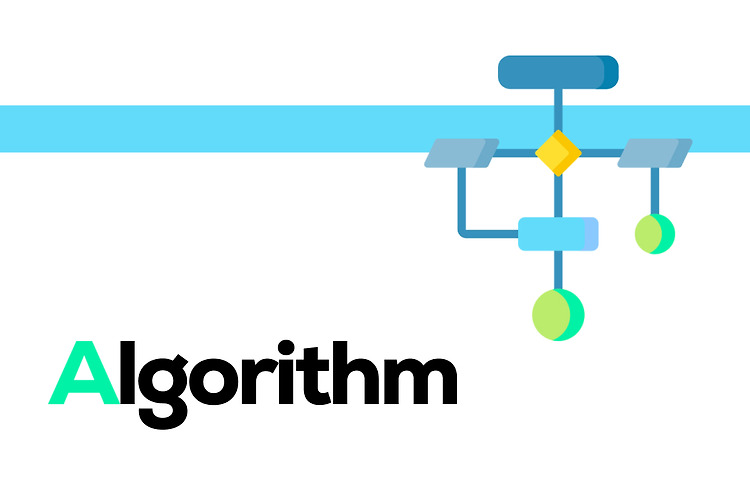
You are given an elliptical shaped land and you are asked to choose n arbitrary points on its boundary. Then you connect all these points with one another with straight lines (that’s n ∗ (n − 1)/2 connections for n points). What is the maximum number of pieces of land you will get by choosing the points on the boundary carefully? 주어진 타원형 땅에서 n개의 임의의 점을 경계선 위에 선택하고, 이러한 점들을 직선으로 서로 연결합니다 (n * (n ..
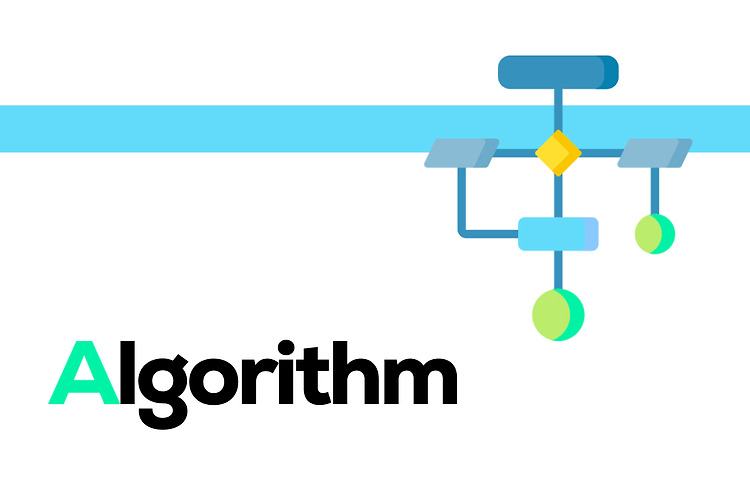
Recall the definition of the Fibonacci numbers: Given two numbers a and b, calculate how many Fibonacci numbers are in the range [a, b]. Input The input contains several test cases. Each test case consists of two non-negative integer numbers a and b. Input is terminated by a = b = 0. Otherwise, a ≤ b ≤ 10100. The numbers a and b are given with no superfluous leading zeros. Output For each test c..
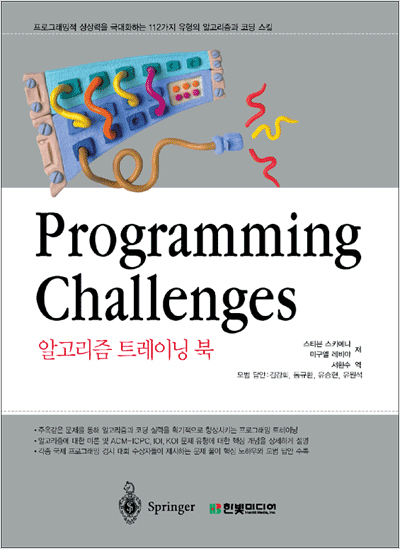
For 10 > N > 2 numbers we form N ∗(N −1)/2 sums by adding every pair of the numbers. Your task is to find the N numbers given the sums. 10 > N > 2인 숫자들을 사용하여 N*(N-1)/2개의 합을 만듭니다. 이때 각 숫자들의 모든 조합을 더해서 합을 구합니다. 주어진 합을 기반으로 N개의 숫자를 찾는 것이 당신의 과제입니다. Input Each line of input contains N followed by N ∗ (N − 1)/2 integer numbers separated by a space. 각 입력 줄은 N 다음에 N * (N - 1) / 2 개의 정수 숫자가 공백으로 구분되어 나옵..
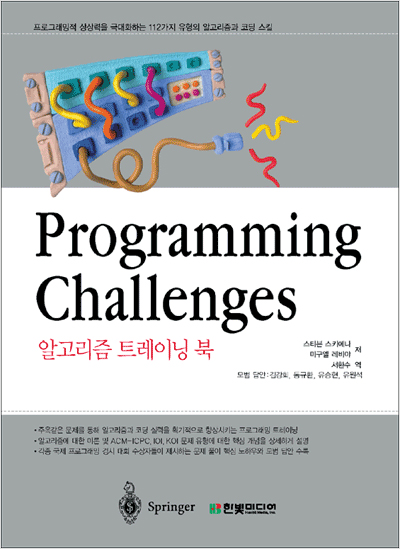
The Stern-Brocot tree is a beautiful way for constructing the set of all nonnegative fractions m/n where m and n are relatively prime. The idea is to start with two fractions ( 0/1 , 1/0 ) and then repeat the following operations as many times as desired: Insert m+m′/n+n′ between two adjacent fractions m/n and m′/n′ . For example, the first step gives us one new entry between 0/1 and 1/0 Stern-B..
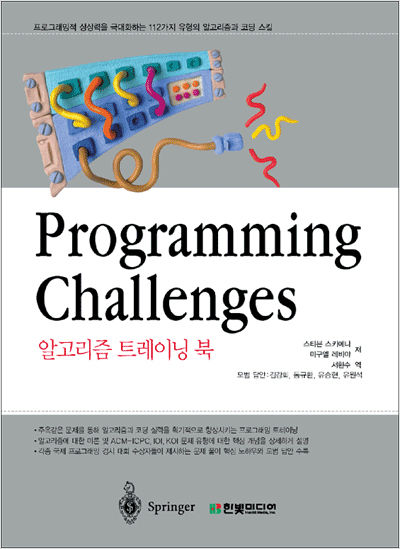
Stan and Ollie play the game of multiplication by multiplying an integer p by one of the numbers 2 to 9. Stan always starts with p = 1, does his multiplication, then Ollie multiplies the number, then Stan and so on. Before a game starts, they draw an integer 1 < n < 4294967295 and the winner is who first reaches p ≥ n. Stan과 Ollie가 정수 p 에 2 이상 9이하의 수 가운데 하나를 곱하는 곱하기 게임을 한다. 항상 가장 먼저 게임을 시작하는 것은 ..
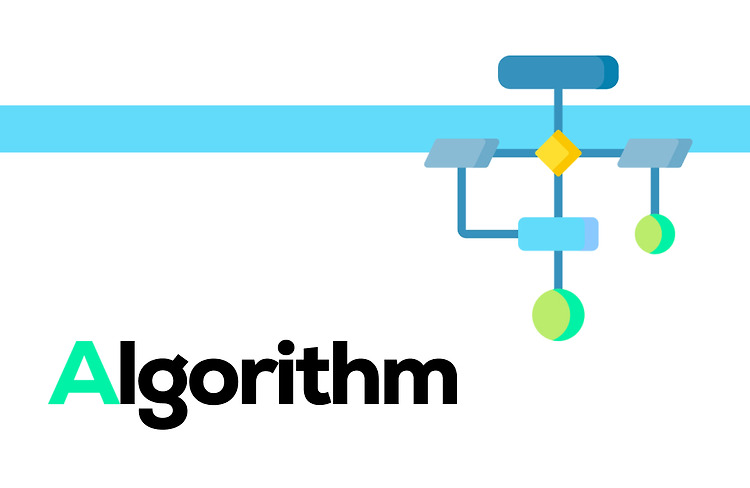
힙 정렬에서는 최소 힙(Min Heap)과 최대 힙(Max Heap) 두 가지 유형의 힙을 사용할 수 있습니다. 다음으로 각각의 힙에 대해 설명해드리겠습니다: 최소 힙(Min Heap): 최소 힙은 부모 노드가 자식 노드보다 작거나 같은 값을 갖는 이진 트리입니다. 이 힙에서는 가장 작은 값이 루트 노드에 위치합니다. 최소 힙을 사용하면 힙에서 가장 작은 값에 빠르게 접근할 수 있습니다. 최대 힙(Max Heap): 최대 힙은 부모 노드가 자식 노드보다 크거나 같은 값을 갖는 이진 트리입니다. 이 힙에서는 가장 큰 값이 루트 노드에 위치합니다. 최대 힙을 사용하면 힙에서 가장 큰 값에 빠르게 접근할 수 있습니다. 힙 정렬에서는 일반적으로 최대 힙을 사용하여 정렬을 수행합니다. 정렬할 배열을 최대 힙으로 ..
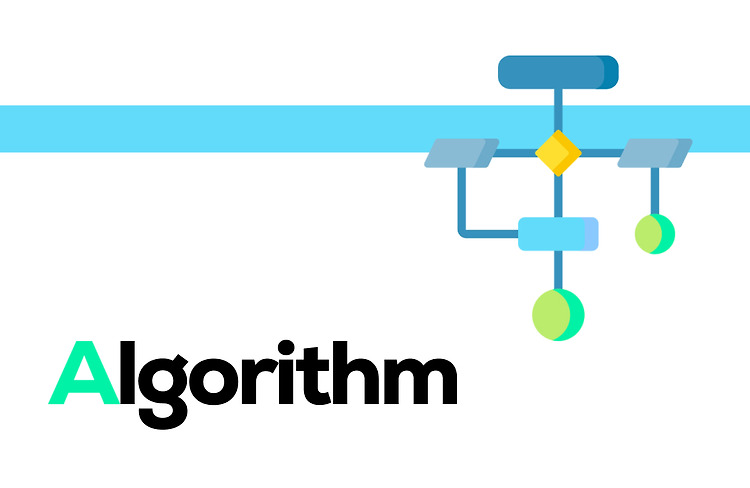
퀵 정렬(Quick Sort)은 분할 정복(divide and conquer) 방식을 사용하여 배열을 정렬하는 알고리즘입니다. 주어진 배열을 기준값(pivot)을 기준으로 작은 값은 왼쪽으로, 큰 값은 오른쪽으로 분할하고, 분할된 부분 배열에 대해 재귀적으로 정렬을 수행합니다. 그림으로 설명을 드리겠습니다. 그림1 : 정렬하고자 하는 배열을 맨 오른쪽 끝을 기준으로 잡아서 새로 생성한 배열 2개(좌, 우)에 기준으로 잡은 배열값(맨 마지막 열 값 10부터)보다 작으면 좌측 배열에 넣고, 크면 우측 배열에 넣습니다. 이 부분을 각 좌측 배열과, 우측 배열을 재귀함수를 이용하여 다시 좌우측으로 정렬하는 작업을 반복합니다. 그림2 : 더이상 좌, 우측 배열로 나눌 데이터가 없는 경우 회귀를 통해서 각 재귀함수..
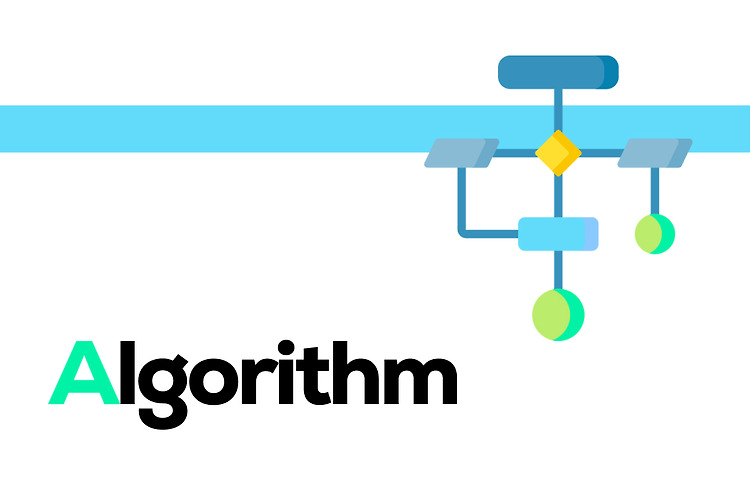
삽입 정렬(Insertion Sort)은 간단한 정렬 알고리즘 중 하나로, 배열을 정렬된 부분과 정렬되지 않은 부분으로 나누고, 정렬되지 않은 부분의 요소를 정렬된 부분에 적절한 위치에 삽입하는 방식으로 동작합니다. 아래는 JavaScript로 구현된 삽입 정렬 알고리즘의 예시입니다 function insertionSort(arr) { const n = arr.length; for (let i = 1; i = 0 && arr[j] > current) { arr[j + 1] = arr[j]; j--; } arr[j + 1] = current; } return arr; } // 사용 예시 const ar..
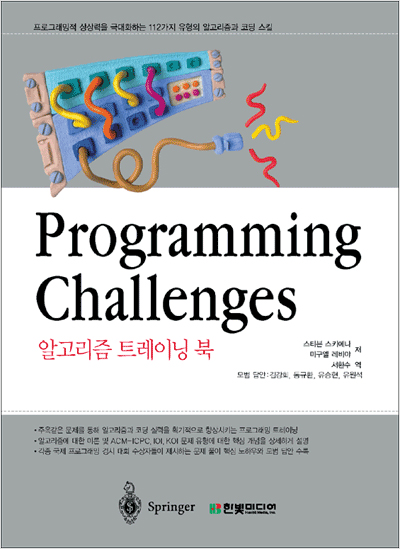
문제 : 2나 5로 나눌 수 없는 0 이상 10,000 이하의 정수 n이 주어졌는데, n의 배수 중에는 10진수로 표기 했을때 모든 자리수가 1인 것이 있다. 그러한 n의 배수중에서 가장 작은 것은 몇자리 수 일까? let integerArray = new Array(10000).fill(0) integerArray = integerArray.reduce((ac, e, i, array) => { if (i%2 != 0 && i%5 != 0) { ac.push(i); } return ac }, []).filter(e => e != 1); integerArray.forEach(function (e) { calculator(e); }) function calculator(e) { let result = [];..
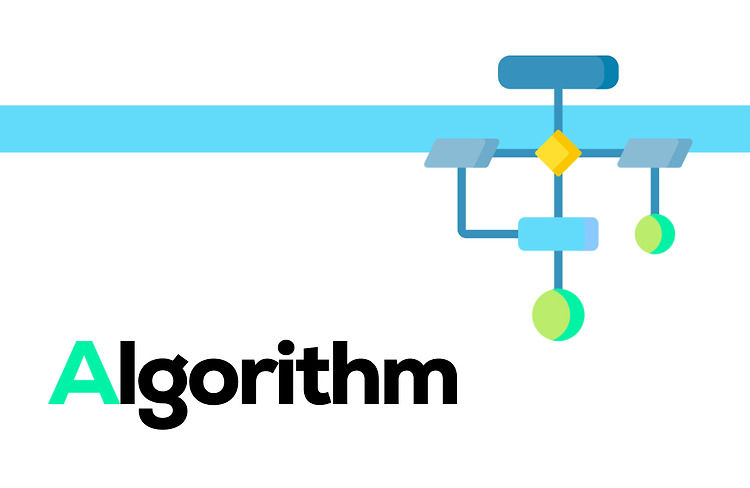
알고리즘은 시간의 복잡도와 공간의 복잡도 두개를 분석하는 영역이 다르다 시간 복잡도(Time complexity)는 알고리즘이 실행되는 동안 소요되는 시간의 측정 공간 복잡도(Space complexity)는 알고리즘이 실행되는 동안 소요되는 메모리 공간의 측정 시간 복잡도는 알고리즘이 입력 크기에 따라 소요되는 시간의 증가율을 나타냅니다. 일반적으로 실행되는 연산 횟수에 대한 함수로 표현됩니다. 시간 복잡도는 알고리즘의 성능을 측정하고, 알고리즘 간의 비교 및 선택에 사용됩니다. 빅 오 표기법과 같은 점근적 표기법을 사용하여 알고리즘의 시간 복잡도를 표기합니다. 공간 복잡도는 알고리즘이 실행되는 동안 필요한 메모리 공간의 양을 측정합니다. 주로 추가적인 배열, 변수, 데이터 구조 등의 메모리 사용량을 ..